Need More Help Getting Started?
A simple guide to access your company's data!
ShippingTree's API provides critical information to filter and view company metrics. Our app provides many standardized reports for products, order lists, inventory, billing and many others, but our API gives you more control over the types of data you want visibility into.
Please Note: The API is only accessible after you have completed the onboarding process with customer service.
Walkthrough
- Once your company has been successfully created an API Key is automatically generated for you. You can see this API Key in your company settings.
- Located near your API key is a link to our API docs. This is where you will find all necessary API calls to access your company's data.
- The link attached in part 2 shows you an example API call. Let's walk through the example here.
- Select the language you wish to make API calls in.
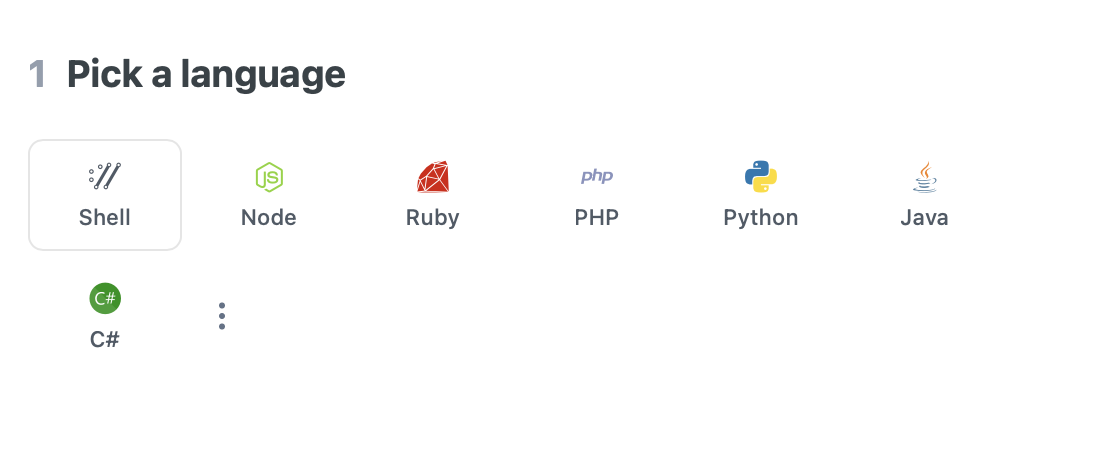
API Code Selection
- Below is the same API key mentioned in part 1 (an actual API Key will be shown in place of YOUR_API_KEY). This will be used to access Shipping Tree's API.
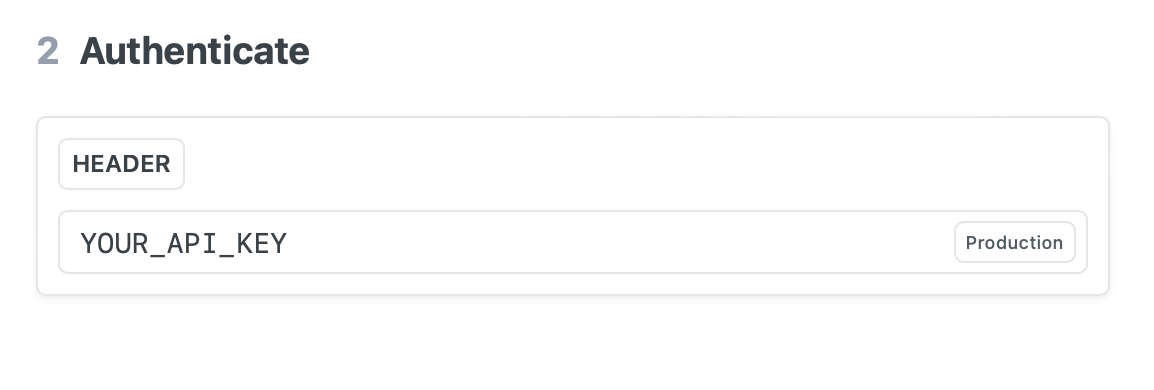
-
Let's run through an example! Below we are using the Python requests library to grab a list of orders. Try ReadMe's interface to test this API call. The interface has a few key features.
-
Your Request History
- Area to easily view how many calls were made in a certain time period, as well as if the calls were successful. You can view status codes here
-
Query Params
-
Query params allow for you to make more specific API calls. For example, we added sku="banana" in the search query param which will now only include orders that have the product "banana". The request URL will automatically update by appending your new query param to the end of the URL.
url = "http://app.shippingtree.co/api/orders/?search=sku%253D%2522banana%2522"
-
-
Request
- As mentioned in query params, when you updated the request, the code on the right hand side will automatically be updated. You can copy this code directly from the ReadMe interface to test yourself or you can run it directly on the ReadMe website.
-
Response
- The response is the outcome of the request. In the below example, you can see the request received a status = 401 due to authentication credentials not being provided. If this occurs, double check your API key and make sure it matches the one on Shipping Tree. If the API keys match, please reach out to customer support to help resolve this issue.
-
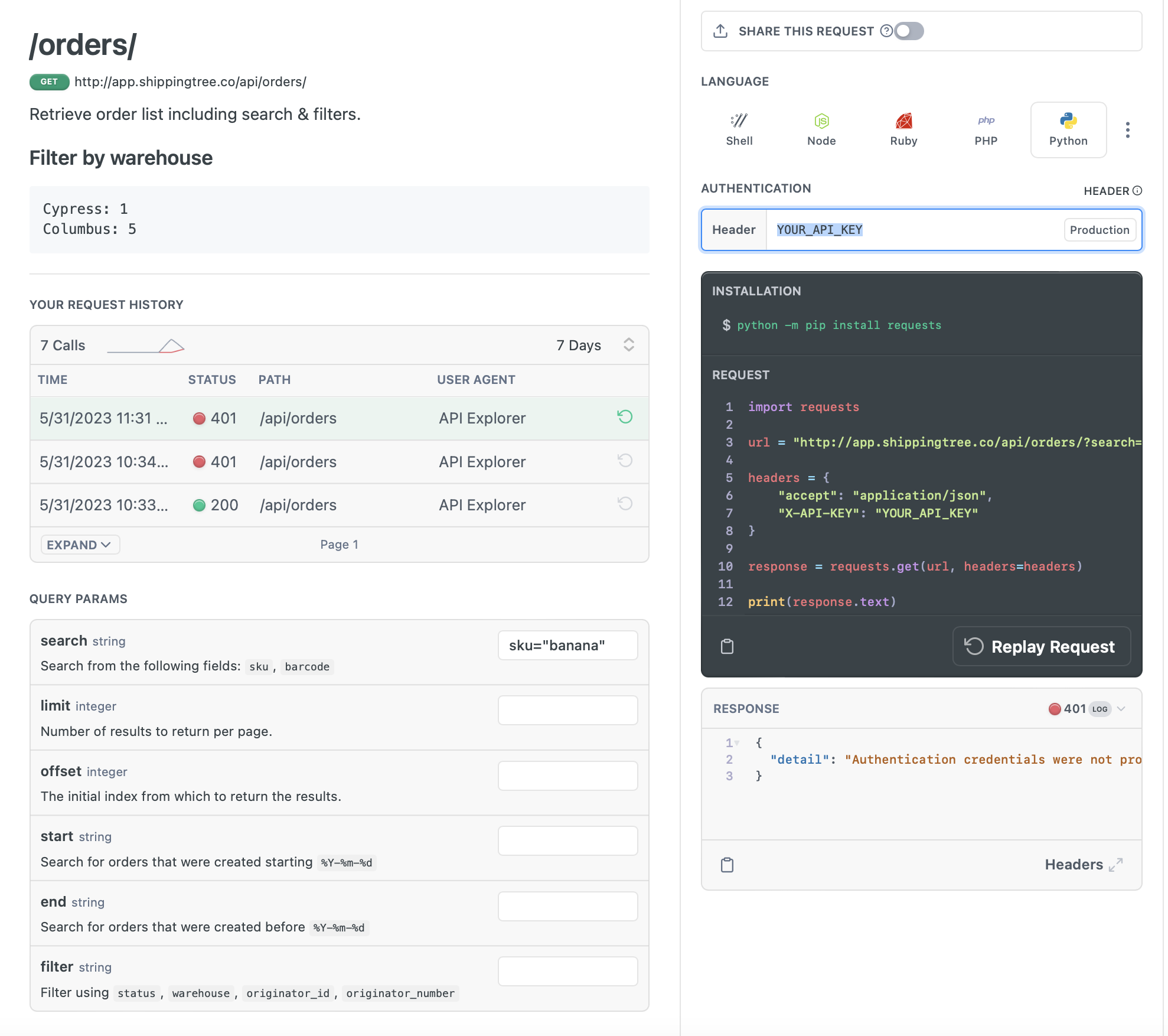
Example API Request
-
Here is a closer look at an example API call.
import requests # Endpoint that allows retrieval of company orders url = "http://app.shippingtree.co/api/orders/?search=sku%253D%2522banana%2522" # Neccessary headers for request # Replace YOUR_API_KEY with API key mentioned above headers = { "accept": "application/json", "X-API-KEY": "<YOUR_API_KEY>" } # Response that will return desired data response = requests.get(url, headers=headers) print(response.text)
-
Below is a successful response from the GET orders API endpoint. You will receive the count of orders returned and a list of orders with related data.
{ "count": 1053, "next": "http://app.shippingtree.co/api/orders/?limit=10&offset=10", "previous": null, "results": [ { "id": 5573669, "status": 200, "items": [ { "sku": "ICE", "quantity": 40, "price": null }, { "sku": "COLDBAG", "quantity": 40, "price": null }, { "sku": "8X8X4", "quantity": 20, "price": null } ], "shipments": [], "inventory_transactions": [ { "id": 44395535, "inventory_item": { "id": 843972, "sku": "8X8X4", "qr_code": "cb314e56-ec13-46f8-8957-91a9c4572e55", "lot_number": null, "manufacture_date": null, "best_before_date": null, "expiry_date": null, "received_date": "2021-10-27", "inventory_type": "production", "created": "2023-05-30T12:54:20.895875-07:00", "updated": "2023-05-30T12:54:20.895889-07:00" }, "adjustment": false, "movement": false, "quantity": -20, "comment": null, "created": "2023-05-30T12:54:44.737116-07:00", "updated": "2023-05-30T12:54:44.737127-07:00" }, { "id": 44395534, "inventory_item": { "id": 843973, "sku": "COLDBAG", "qr_code": "4ac8549d-cb3c-47b5-bc4f-75c564b2af00", "lot_number": null, "manufacture_date": null, "best_before_date": null, "expiry_date": null, "received_date": "2021-10-22", "inventory_type": "production", "created": "2023-05-30T12:54:20.931902-07:00", "updated": "2023-05-30T12:54:20.931913-07:00" }, "adjustment": false, "movement": false, "quantity": -40, "comment": null, "created": "2023-05-30T12:54:44.681122-07:00", "updated": "2023-05-30T12:54:44.681133-07:00" }, { "id": 44395533, "inventory_item": { "id": 843974, "sku": "ICE", "qr_code": "ebeea69d-76a9-43a1-b94a-f14a2e0d0933", "lot_number": null, "manufacture_date": null, "best_before_date": null, "expiry_date": null, "received_date": "2022-05-16", "inventory_type": "production", "created": "2023-05-30T12:54:20.963323-07:00", "updated": "2023-05-30T12:54:20.963335-07:00" }, "adjustment": false, "movement": false, "quantity": -40, "comment": null, "created": "2023-05-30T12:54:44.625115-07:00", "updated": "2023-05-30T12:54:44.625126-07:00" } ], "email": "[email protected]", "originator_id": null, "shipping_name": "Internal Project Order", "shipping_company": null, "shipping_address_1": "10731 Walker street", "shipping_address_2": "Suite B", "shipping_city": "Cypress", "shipping_state": "CA", "shipping_country": "US", "shipping_zip": "90630-4757", "return_address_1": "10731 Walker street", "return_address_2": "Suite B", "return_city": "Cypress", "return_company": "ShippingTree", "return_name": "Cypress", "return_country": "US", "return_phone": "1 800-728-9984", "return_email": "[email protected]", "return_state": "CA", "return_zip": "90630-4757", "public_note": null, "b_to_b": false, "ship_by_date": null, "ship_before": null, "ship_after": null, "maturation": "2023-05-30T13:09:44.041172-07:00", "fulfilled": null, "shipped": "2023-05-30T12:56:10.710415-07:00", "delivered": null, "shipping_phone": "1 800-728-9984", "shop_shipping_method_text": null, "shipping_customer_pickup": true, "use_wholesale_prices": false, "insure": false, "insurance_value": null, "require_signature": false, "saturday_delivery": false, "gift": false, "address_verified": false, "require_adult_signature": false, "contains_alcohol": false, "created": "2023-05-30T12:54:44.041615-07:00", "updated": "2023-05-30T12:56:10.712812-07:00" }, ] }
Thanks for following along! If you have any questions please reach out using our discussion forum.
Updated 2 months ago